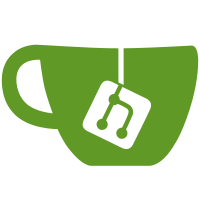
Before this change, when Arduino was linking the elf binary, it added all objects and archives (libraries/plugins) to a long linker command line. This would sometimes cause issues due to the linker's gargabe collection feature. When one library A referenced a symbol from a library B but library A appeared after B in the linker command line, the referenced symbol was in some cases garbage collected as to the linker it appeared to be unreferenced. To understand this better it is important to know that the linker processes objects and archives one by one in the order of their appearance at the command line. For every object or archive encountered it runs garbage collection individually. That's why symbols might get discarded although an object or archives that is encountered later on while processing the linker command line might have referenced it. To fix this problem this commit divides up the linker process into two steps. First, all objects and archives are combined in a large .a archive. Then, this archive is passed to the original linker command line, thereby replacing the original set of objects and archives. The final link now sees all symbols together. Thus, it is able to determine the true interdependencies and do a proper garbage collection without accidentally generating unresolved symbols. With this fix, the order of appearance of include directives in the sketch file that formerly had an influence on the link order (as Arduino passes library archives to the linker in the order of the appearance of the matching includes in the sketch), can now be savely chosen arbitrarily. To erase the joined archive file that is generated from all objects and archives, we have to use different solutions on Linux/macOS and Windows. This is done via defining tools.rm.cmd differently for those platforms. Signed-off-by: Florian Fleissner <florian.fleissner@inpartik.de>
160 lines
9.0 KiB
Plaintext
160 lines
9.0 KiB
Plaintext
|
|
# Arduino AVR Core and platform.
|
|
# ------------------------------
|
|
#
|
|
# For more info:
|
|
# https://github.com/arduino/Arduino/wiki/Arduino-IDE-1.5-3rd-party-Hardware-specification
|
|
|
|
name=Keyboardio Keyboards
|
|
version=1.6.11
|
|
|
|
# AVR compile variables
|
|
# ---------------------
|
|
|
|
compiler.warning_flags=-w
|
|
compiler.warning_flags.none=-w
|
|
compiler.warning_flags.default=
|
|
compiler.warning_flags.more=-Wall
|
|
compiler.warning_flags.all=-Wall -Wextra
|
|
|
|
# Default "compiler.path" is correct, change only if you want to override the initial value
|
|
compiler.path={runtime.tools.avr-gcc.path}/bin/
|
|
compiler.c.cmd=avr-gcc
|
|
compiler.c.flags=-c -g -Os {compiler.warning_flags} -std=gnu11 -ffunction-sections -fdata-sections -MMD
|
|
compiler.c.elf.flags={compiler.warning_flags} -Os -Wl,--gc-sections
|
|
compiler.c.elf.flags_join_archives=rcT
|
|
compiler.c.elf.cmd=avr-gcc
|
|
compiler.S.flags=-c -g -x assembler-with-cpp
|
|
compiler.cpp.cmd=avr-g++
|
|
compiler.cpp.flags=-c -g -Os {compiler.warning_flags} -std=gnu++11 -fno-exceptions -ffunction-sections -fdata-sections -fno-threadsafe-statics -MMD
|
|
compiler.ar.cmd=avr-ar
|
|
compiler.ar.flags=rcs
|
|
compiler.objcopy.cmd=avr-objcopy
|
|
compiler.objcopy.eep.flags=-O ihex -j .eeprom --set-section-flags=.eeprom=alloc,load --no-change-warnings --change-section-lma .eeprom=0
|
|
compiler.elf2hex.flags=-O ihex -R .eeprom
|
|
compiler.elf2hex.cmd=avr-objcopy
|
|
compiler.ldflags=
|
|
compiler.size.cmd=avr-size
|
|
|
|
# This can be overridden in boards.txt
|
|
build.extra_flags=
|
|
|
|
# These can be overridden in platform.local.txt
|
|
compiler.c.extra_flags=
|
|
compiler.c.elf.extra_flags=
|
|
compiler.S.extra_flags=
|
|
compiler.cpp.extra_flags=-Woverloaded-virtual -Wno-unused-parameter -Wno-unused-variable -Wno-ignored-qualifiers
|
|
compiler.ar.extra_flags=
|
|
compiler.objcopy.eep.extra_flags=
|
|
compiler.elf2hex.extra_flags=
|
|
|
|
# AVR compile patterns
|
|
# --------------------
|
|
|
|
## Compile c files
|
|
recipe.c.o.pattern="{compiler.path}{compiler.c.cmd}" {compiler.c.flags} -mmcu={build.mcu} -DF_CPU={build.f_cpu} -DARDUINO={runtime.ide.version} -DARDUINO_{build.board} -DARDUINO_ARCH_{build.arch} {compiler.c.extra_flags} {build.extra_flags} {includes} "{source_file}" -o "{object_file}"
|
|
|
|
## Compile c++ files
|
|
recipe.cpp.o.pattern="{compiler.path}{compiler.cpp.cmd}" {compiler.cpp.flags} -mmcu={build.mcu} -DF_CPU={build.f_cpu} -DARDUINO={runtime.ide.version} -DARDUINO_{build.board} -DARDUINO_ARCH_{build.arch} {compiler.cpp.extra_flags} {build.extra_flags} {includes} "{source_file}" -o "{object_file}"
|
|
|
|
## Compile S files
|
|
recipe.S.o.pattern="{compiler.path}{compiler.c.cmd}" {compiler.S.flags} -mmcu={build.mcu} -DF_CPU={build.f_cpu} -DARDUINO={runtime.ide.version} -DARDUINO_{build.board} -DARDUINO_ARCH_{build.arch} {compiler.S.extra_flags} {build.extra_flags} {includes} "{source_file}" -o "{object_file}"
|
|
|
|
## Create archives
|
|
# archive_file_path is needed for backwards compatibility with IDE 1.6.5 or older, IDE 1.6.6 or newer overrides this value
|
|
archive_file_path={build.path}/{archive_file}
|
|
recipe.ar.pattern="{compiler.path}{compiler.ar.cmd}" {compiler.ar.flags} {compiler.ar.extra_flags} "{archive_file_path}" "{object_file}"
|
|
|
|
################################################################################
|
|
## PLEASE NOTE: The way we link our elf binary significantly differs from the
|
|
## way that Arduino handles things by default.
|
|
## The original linker command has been split up into three
|
|
## steps (recipe.c.combine.pattern, recipe.hooks.linking.postlink.1.pattern
|
|
## and recipe.hooks.linking.postlink.2.pattern).
|
|
## This is necessary to prevent link errors reporting unresolved symbols
|
|
## due to order dependencies of libraries and objects appearing
|
|
## in the linker command line.
|
|
##
|
|
## CHANGED WRT DEFAULT: Generate a large .a archive to prevent link order issues with garbage collection
|
|
recipe.c.combine.pattern="{compiler.path}/{compiler.ar.cmd}" {compiler.c.elf.flags_join_archives} "{build.path}/{build.project_name}_joined.a" {object_files} "{build.path}/{archive_file}"
|
|
##
|
|
## NEWLY INTRODUCED: Link with global garbage collection (considering all
|
|
## objects and libraries together).
|
|
recipe.hooks.linking.postlink.1.pattern="{compiler.path}{compiler.c.elf.cmd}" {compiler.c.elf.flags} -mmcu={build.mcu} {compiler.c.elf.extra_flags} -o "{build.path}/{build.project_name}.elf" "{build.path}/{build.project_name}_joined.a" "-L{build.path}" -lm
|
|
##
|
|
## NEWLY INTRODUCED: Removing the joined library is required to avoid malformed archives that
|
|
## would otherwise result when updating a pre-existing
|
|
## joined archive file during a subsequent firmware build.
|
|
##
|
|
recipe.hooks.linking.postlink.2.pattern={tools.rm.cmd} "{build.path}/{build.project_name}_joined.a"
|
|
################################################################################
|
|
|
|
## Create output files (.eep and .hex)
|
|
recipe.objcopy.eep.pattern="{compiler.path}{compiler.objcopy.cmd}" {compiler.objcopy.eep.flags} {compiler.objcopy.eep.extra_flags} "{build.path}/{build.project_name}.elf" "{build.path}/{build.project_name}.eep"
|
|
recipe.objcopy.hex.pattern="{compiler.path}{compiler.elf2hex.cmd}" {compiler.elf2hex.flags} {compiler.elf2hex.extra_flags} "{build.path}/{build.project_name}.elf" "{build.path}/{build.project_name}.hex"
|
|
|
|
## Save hex
|
|
recipe.output.tmp_file={build.project_name}.hex
|
|
recipe.output.save_file={build.project_name}.{build.variant}.hex
|
|
|
|
## Compute size
|
|
recipe.size.pattern="{compiler.path}{compiler.size.cmd}" -A "{build.path}/{build.project_name}.elf"
|
|
recipe.size.regex=^(?:\.text|\.data|\.bootloader)\s+([0-9]+).*
|
|
recipe.size.regex.data=^(?:\.data|\.bss|\.noinit)\s+([0-9]+).*
|
|
recipe.size.regex.eeprom=^(?:\.eeprom)\s+([0-9]+).*
|
|
|
|
## Preprocessor
|
|
preproc.includes.flags=-w -x c++ -M -MG -MP
|
|
recipe.preproc.includes="{compiler.path}{compiler.cpp.cmd}" {compiler.cpp.flags} {preproc.includes.flags} -mmcu={build.mcu} -DF_CPU={build.f_cpu} -DARDUINO={runtime.ide.version} -DARDUINO_{build.board} -DARDUINO_ARCH_{build.arch} {compiler.cpp.extra_flags} {build.extra_flags} {includes} "{source_file}"
|
|
|
|
preproc.macros.flags=-w -x c++ -E -CC
|
|
recipe.preproc.macros="{compiler.path}{compiler.cpp.cmd}" {compiler.cpp.flags} {preproc.macros.flags} -mmcu={build.mcu} -DF_CPU={build.f_cpu} -DARDUINO={runtime.ide.version} -DARDUINO_{build.board} -DARDUINO_ARCH_{build.arch} {compiler.cpp.extra_flags} {build.extra_flags} {includes} "{source_file}" -o "{preprocessed_file_path}"
|
|
|
|
# AVR Uploader/Programmers tools
|
|
# ------------------------------
|
|
|
|
tools.avrdude.path={runtime.tools.avrdude.path}
|
|
tools.avrdude.cmd.path={path}/bin/avrdude
|
|
tools.avrdude.config.path={path}/etc/avrdude.conf
|
|
|
|
tools.avrdude.upload.params.verbose=-v
|
|
tools.avrdude.upload.params.quiet=-q -q
|
|
tools.avrdude.upload.params.noverify=-V
|
|
tools.avrdude.upload.pattern="{cmd.path}" "-C{config.path}" {upload.verbose} {upload.verify} -p{build.mcu} -c{upload.protocol} -P{serial.port} -b{upload.speed} -D "-Uflash:w:{build.path}/{build.project_name}.hex:i"
|
|
|
|
tools.avrdude.program.params.verbose=-v
|
|
tools.avrdude.program.params.quiet=-q -q
|
|
tools.avrdude.program.params.noverify=-V
|
|
tools.avrdude.program.pattern="{cmd.path}" "-C{config.path}" {program.verbose} {program.verify} -p{build.mcu} -c{protocol} {program.extra_params} "-Uflash:w:{build.path}/{build.project_name}.hex:i"
|
|
|
|
tools.avrdude.erase.params.verbose=-v
|
|
tools.avrdude.erase.params.quiet=-q -q
|
|
tools.avrdude.erase.pattern="{cmd.path}" "-C{config.path}" {erase.verbose} -p{build.mcu} -c{protocol} {program.extra_params} -e -Ulock:w:{bootloader.unlock_bits}:m -Uefuse:w:{bootloader.extended_fuses}:m -Uhfuse:w:{bootloader.high_fuses}:m -Ulfuse:w:{bootloader.low_fuses}:m
|
|
|
|
tools.avrdude.bootloader.params.verbose=-v
|
|
tools.avrdude.bootloader.params.quiet=-q -q
|
|
tools.avrdude.bootloader.pattern="{cmd.path}" "-C{config.path}" {bootloader.verbose} -p{build.mcu} -c{protocol} {program.extra_params} "-Uflash:w:{runtime.platform.path}/bootloaders/{bootloader.file}:i" -Ulock:w:{bootloader.lock_bits}:m
|
|
|
|
tools.avrdude_remote.upload.pattern=/usr/bin/run-avrdude /tmp/sketch.hex {upload.verbose} -p{build.mcu}
|
|
|
|
tools.teensy_loader_cli.cmd.path=teensy_loader_cli
|
|
tools.teensy_loader_cli.upload.params.verbose=-v
|
|
tools.teensy_loader_cli.upload.params.quiet=
|
|
tools.teensy_loader_cli.upload.noverify=
|
|
tools.teensy_loader_cli.upload.pattern="{cmd.path}" {upload.verbose} --mcu=atmega32u4 -w -v "{build.path}/{build.project_name}.hex"
|
|
|
|
tools.dfu-programmer.cmd.path=dfu-programmer
|
|
tools.dfu-programmer.upload.params.verbose=-v
|
|
tools.dfu-programmer.upload.params.quiet=
|
|
tools.dfu-programmer.upload.noverify=
|
|
tools.dfu-programmer.upload.pattern=/bin/sh -c '"{cmd.path}" atmega32u4 erase && "{cmd.path}" atmega32u4 flash "{build.path}/{build.project_name}.hex" && "{cmd.path}" atmega32u4 start'
|
|
|
|
tools.rm.cmd=rm -f
|
|
tools.rm.cmd.windows=powershell.exe Remove-Item -LiteralPath
|
|
|
|
# USB Default Flags
|
|
# Default blank usb manufacturer will be filled in at compile time
|
|
# - from numeric vendor ID, set to Unknown otherwise
|
|
build.usb_manufacturer="Unknown"
|
|
build.usb_flags=-DUSB_VID={build.vid} -DUSB_PID={build.pid} '-DUSB_MANUFACTURER={build.usb_manufacturer}' '-DUSB_PRODUCT={build.usb_product}'
|