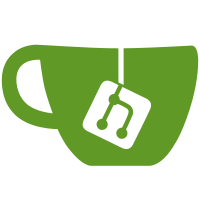
This makes the build much simpler as the FQBN can now e.g. be given as keyboardio:virtual:model01 and the build process will automatically find the virtual arduino core in virtual/cores/arduino Signed-off-by: Florian Fleissner <florian.fleissner@inpartik.de>
55 lines
1.3 KiB
C++
55 lines
1.3 KiB
C++
#pragma once
|
|
|
|
#include "Stream.h"
|
|
#include <stdio.h>
|
|
|
|
class HardwareSerial : public Stream {
|
|
public:
|
|
HardwareSerial();
|
|
void begin(unsigned long baud) {
|
|
begin(baud, 0x06);
|
|
}
|
|
void begin(unsigned long, uint8_t);
|
|
void end();
|
|
virtual int available();
|
|
virtual int peek();
|
|
virtual int read();
|
|
virtual int availableForWrite();
|
|
virtual void flush();
|
|
virtual size_t write(uint8_t);
|
|
// we keep these four write()s the same as the default Arduino core
|
|
inline size_t write(unsigned long n) {
|
|
return write((uint8_t)n);
|
|
}
|
|
inline size_t write(long n) {
|
|
return write((uint8_t)n);
|
|
}
|
|
inline size_t write(unsigned int n) {
|
|
return write((uint8_t)n);
|
|
}
|
|
inline size_t write(int n) {
|
|
return write((uint8_t)n);
|
|
}
|
|
using Print::write; // write(str) and write(buf, size)
|
|
operator bool() {
|
|
return true;
|
|
}
|
|
private:
|
|
static unsigned serialNumber;
|
|
FILE* out;
|
|
};
|
|
// The default Arduino core only provides each of these HardwareSerial objects if
|
|
// various things are #defined. We always provide them for virtual hardware.
|
|
extern HardwareSerial Serial;
|
|
#define HAVE_HWSERIAL0
|
|
extern HardwareSerial Serial1;
|
|
#define HAVE_HWSERIAL1
|
|
extern HardwareSerial Serial2;
|
|
#define HAVE_HWSERIAL2
|
|
extern HardwareSerial Serial3;
|
|
#define HAVE_HWSERIAL3
|
|
// end HardwareSerial
|
|
|
|
extern void serialEventRun(void) __attribute__((weak));
|
|
|